Unraveling the Maximum Difference Between Successive Elements - A Coding Challenge
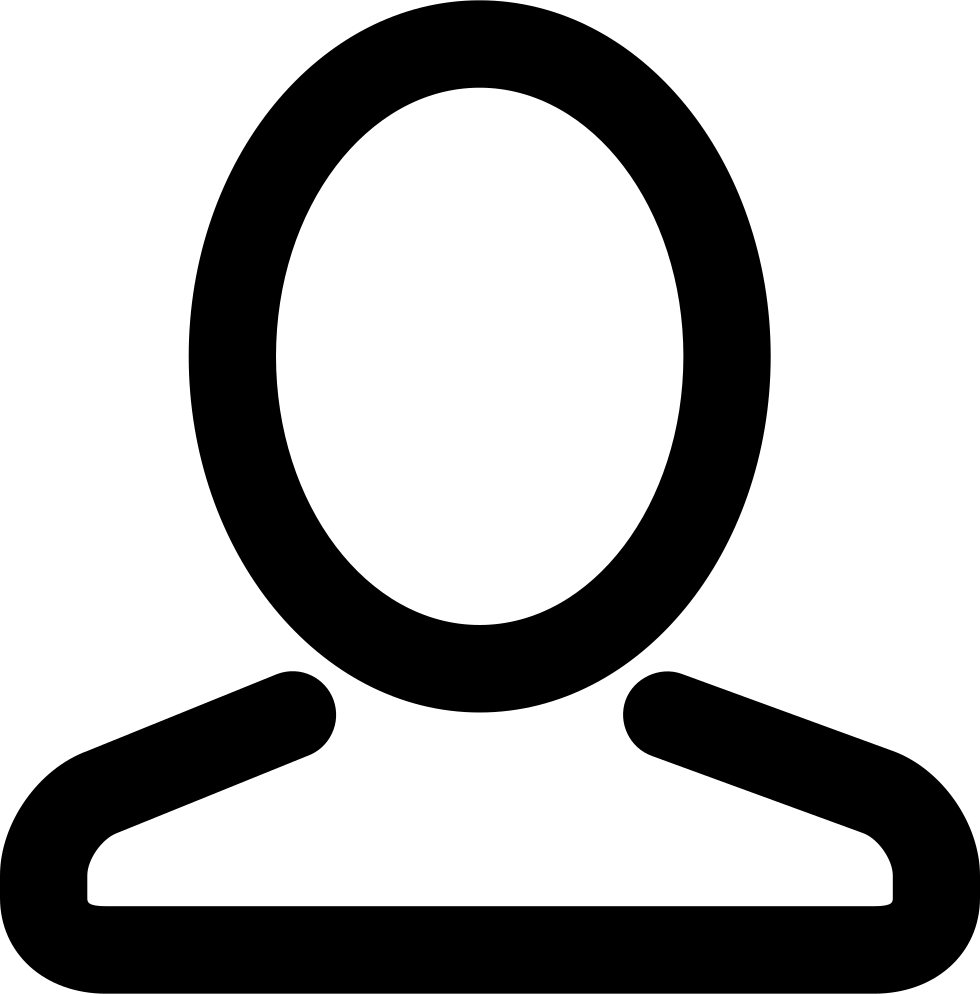
Introduction:
In the realm of coding challenges, there are captivating problems that put our analytical skills to the test. In this blog, we will delve into the challenge of finding the maximum difference between two successive elements in a sorted version of an integer array. We will explore the problem statement, discuss the approach, and provide a comprehensive implementation in both Java and Python.
Problem Statement:
Given an integer array nums, our objective is to find the maximum difference between two successive elements in the sorted form of the array.
Example:
Consider the array [6, 10, 3, 9, 2]:
- After sorting the array in ascending order, we obtain [2, 3, 6, 9, 10].
- The maximum difference between two successive elements is 9 - 6 = 3. Hence, the maximum difference in this case is 3.
Approach:
To solve this problem, we need to sort the given array in ascending order and then calculate the difference between each pair of successive elements. We will track the maximum difference as we iterate through the sorted array.
- Sort the array in ascending order.
- Iterate through the sorted array and calculate the difference between each successive pair of elements.
- Track the maximum difference found during the iteration.
- Return the maximum difference as the result.
Java Implementation:
import java.util.Arrays;
public class MaximumSuccessiveDifference {
public static int maxSuccessiveDifference(int[] nums) {
Arrays.sort(nums);
int maxDiff = 0;
for (int i = 1; i < nums.length; i++) {
int diff = nums[i] - nums[i - 1];
maxDiff = Math.max(maxDiff, diff);
}
return maxDiff;
}
public static void main(String[] args) {
int[] nums = {6, 10, 3, 9, 2};
int maxDiff = maxSuccessiveDifference(nums);
System.out.println("Maximum Successive Difference: " + maxDiff);
}
}
Python Implementation:
def max_successive_difference(nums):
nums.sort()
max_diff = 0
for i in range(1, len(nums)):
diff = nums[i] - nums[i - 1]
max_diff = max(max_diff, diff)
return max_diff
nums = [6, 10, 3, 9, 2]
max_diff = max_successive_difference(nums)
print("Maximum Successive Difference:", max_diff)
Conclusion:
Solving the challenge of finding the maximum difference between two successive elements in a sorted array allows us to flex our problem-solving muscles. By sorting the array and iterating through the sorted version, we can efficiently calculate the maximum difference. The implementations in Java and Python demonstrate the elegance and simplicity of the solution. Armed with this newfound knowledge, you can confidently tackle similar coding challenges and elevate your programming skills.