Unraveling the Longest Substring Without Repeating Characters Puzzle
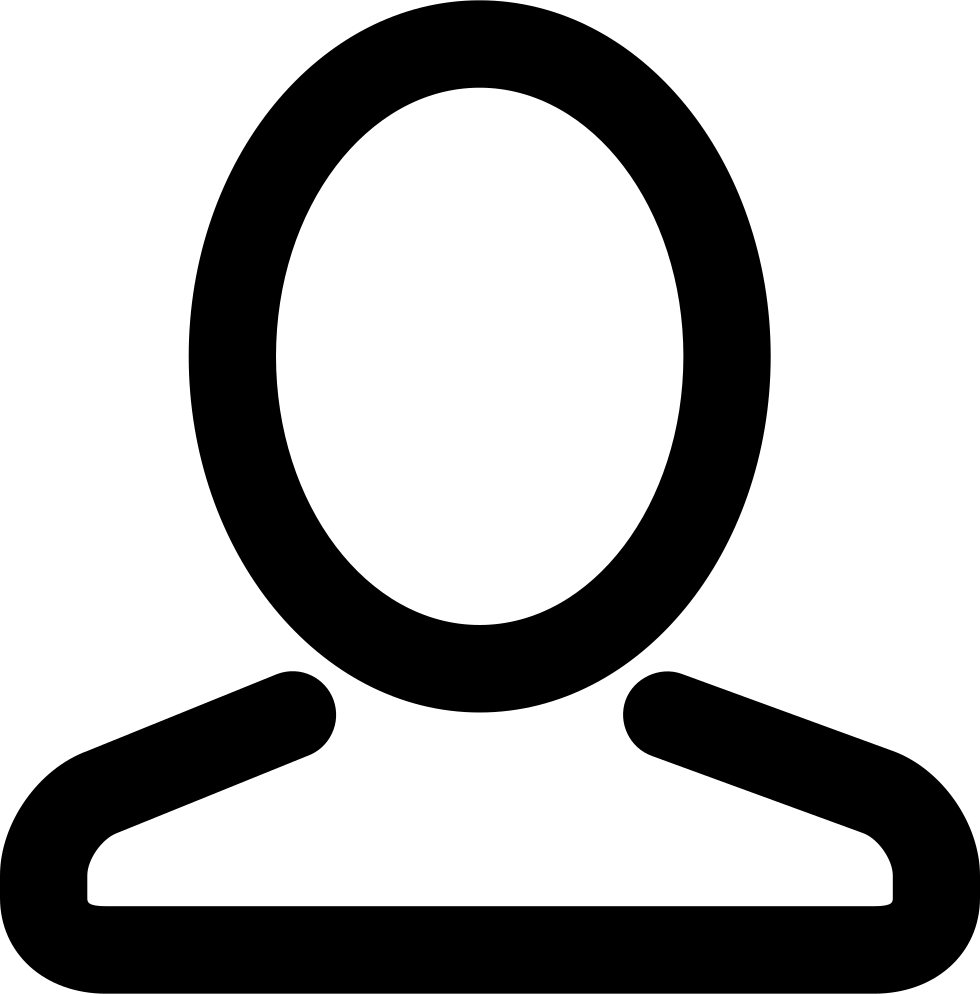
In the realm of string manipulation, the challenge of finding the longest substring without repeating characters stands as a captivating puzzle. In this blog, we will delve into the problem of identifying the longest substring in a given string that does not contain any repeating characters. We will explore the problem statement, discuss potential approaches, and provide detailed implementations in both Java and Python.
Problem Statement:
Given a string, our goal is to find the length of the longest substring that does not contain any repeating characters. The substring can be of any length and must consist of consecutive characters from the original string.
Example:
Consider the string "abcabcbb". The longest substring without repeating characters in this case is "abc", with a length of 3.
Approach:
To solve the problem of finding the longest substring without repeating characters, we can utilize a sliding window technique. We will maintain a window that grows from the left end of the string, adding characters one by one. If a repeating character is encountered, we will adjust the window by moving the left pointer to the right, ensuring that the window only contains unique characters. We will track the maximum length of the non-repeating substring as we iterate through the string.
- Initialize variables for the maximum length, left pointer, and a set to store unique characters.
- Iterate through the string using a right pointer:
- If the character at the right pointer is not in the set, add it to the set and update the maximum length if necessary.
- If the character at the right pointer is already in the set, remove the character at the left pointer from the set and move the left pointer to the right.
- Repeat step 2 until the right pointer reaches the end of the string.
- Return the maximum length of the non-repeating substring.
Java Implementation:
public class LongestSubstring {
public static int lengthOfLongestSubstring(String s) {
int maxLength = 0;
int left = 0;
Set<Character> uniqueChars = new HashSet<>();
for (int right = 0; right < s.length(); right++) {
char currentChar = s.charAt(right);
while (uniqueChars.contains(currentChar)) {
uniqueChars.remove(s.charAt(left));
left++;
}
uniqueChars.add(currentChar);
maxLength = Math.max(maxLength, right - left + 1);
}
return maxLength;
}
public static void main(String[] args) {
String input = "abcabcbb";
int length = lengthOfLongestSubstring(input);
System.out.println("Length of the longest substring without repeating characters: " + length);
}
}
Python Implementation:
def length_of_longest_substring(s):
max_length = 0
left = 0
unique_chars = set()
for right in range(len(s)):
current_char = s[right]
while current_char in unique_chars:
unique_chars.remove(s[left])
left += 1
unique_chars.add(current_char)
max_length = max(max_length, right - left + 1)
return max_length
input_string = "abcabcbb"
length = length_of_longest_substring(input_string)
print("Length of the longest substring without repeating characters:", length)
Conclusion:
Finding the longest substring without repeating characters is a fascinating problem in string manipulation. By utilizing the sliding window technique, we can efficiently track the non-repeating substring's maximum length. The Java and Python implementations provided in this blog exemplify the elegance and effectiveness of this approach. Armed with this knowledge, you can confidently tackle similar string manipulation challenges and enhance your problem-solving skills.