Unleashing the Power of the Sliding Window Maximum Algorithm
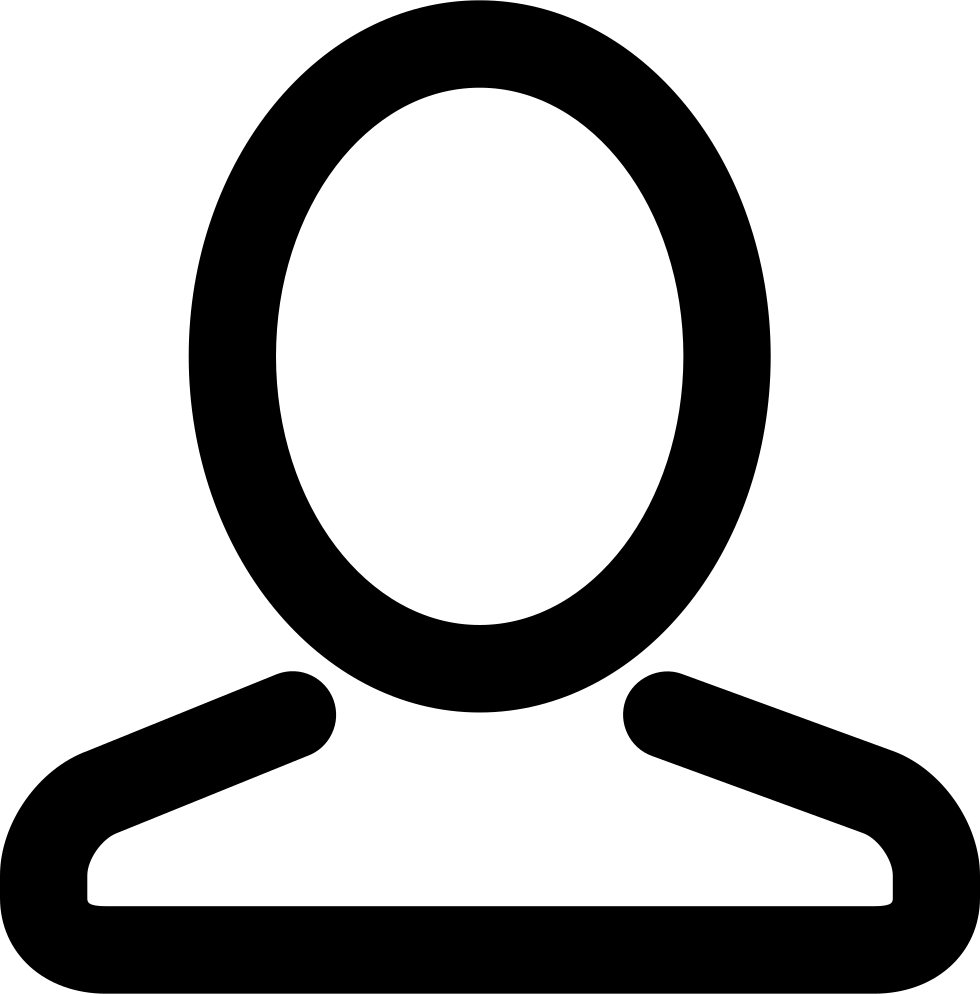
In the realm of algorithmic problem-solving, the sliding window maximum algorithm stands as a powerful technique for efficiently finding the maximum element within a sliding window of elements. In this blog, we will explore the concept of the sliding window maximum, discuss its applications, and provide detailed implementations in both Java and Python.
What is the Sliding Window Maximum?
The sliding window maximum algorithm addresses the challenge of finding the maximum element within a fixed-size window as it slides through an array. It is particularly useful in scenarios where we need to process subarrays or contiguous segments of data efficiently.
Approach:
To solve the sliding window maximum problem, we can utilize a data structure called a deque (double-ended queue) that allows efficient insertions and deletions from both ends. We will maintain the deque such that it stores the indices of elements in descending order of their values. This way, the maximum element will always be at the front of the deque.
- Create an empty deque and iterate through the array.
- For each element, perform the following steps:
- Remove indices from the front of the deque that are outside the current window.
- Remove indices from the back of the deque that correspond to elements smaller than the current element.
- Add the current element's index to the back of the deque.
- If the window size has reached the minimum required size, add the maximum element (at the front of the deque) to the result.
- Return the result containing the sliding window maximum elements.
Java Implementation:
import java.util.ArrayDeque;
import java.util.Deque;
public class SlidingWindowMaximum {
public static int[] maxSlidingWindow(int[] nums, int k) {
if (nums == null || nums.length == 0 || k <= 0)
return new int[0];
int[] result = new int[nums.length - k + 1];
Deque<Integer> deque = new ArrayDeque<>();
for (int i = 0; i < nums.length; i++) {
if (!deque.isEmpty() && deque.peekFirst() < i - k + 1)
deque.pollFirst();
while (!deque.isEmpty() && nums[deque.peekLast()] < nums[i])
deque.pollLast();
deque.offerLast(i);
if (i - k + 1 >= 0)
result[i - k + 1] = nums[deque.peekFirst()];
}
return result;
}
public static void main(String[] args) {
int[] nums = {1, 3, -1, -3, 5, 3, 6, 7};
int k = 3;
int[] slidingWindowMax = maxSlidingWindow(nums, k);
System.out.print("Sliding Window Maximum: ");
for (int num : slidingWindowMax) {
System.out.print(num + " ");
}
}
}
Python Implementation:
from collections import deque
def max_sliding_window(nums, k):
if not nums or k <= 0:
return []
result = []
window = deque()
for i in range(len(nums)):
if window and window[0] < i - k + 1:
window.popleft()
while window and nums[window[-1]] < nums[i]:
window.pop()
window.append(i)
if i - k + 1 >= 0:
result.append(nums[window[0]])
return result
nums = [1, 3, -1, -3, 5, 3, 6, 7]
k = 3
sliding_window_max = max_sliding_window(nums, k)
print("Sliding Window Maximum:", sliding_window_max)
Conclusion:
The sliding window maximum algorithm provides an efficient solution for finding the maximum element within a sliding window of elements in an array. By utilizing the deque data structure and maintaining the maximum element at the front, we can process subarrays and contiguous segments of data with ease. The Java and Python implementations provided in this blog showcase the effectiveness of this approach. Armed with this knowledge, you can confidently solve similar problems that require sliding window operations and elevate your problem-solving skills to new heights.