Unleashing the Maximum Product Difference Between Two Pairs - A Coding Challenge
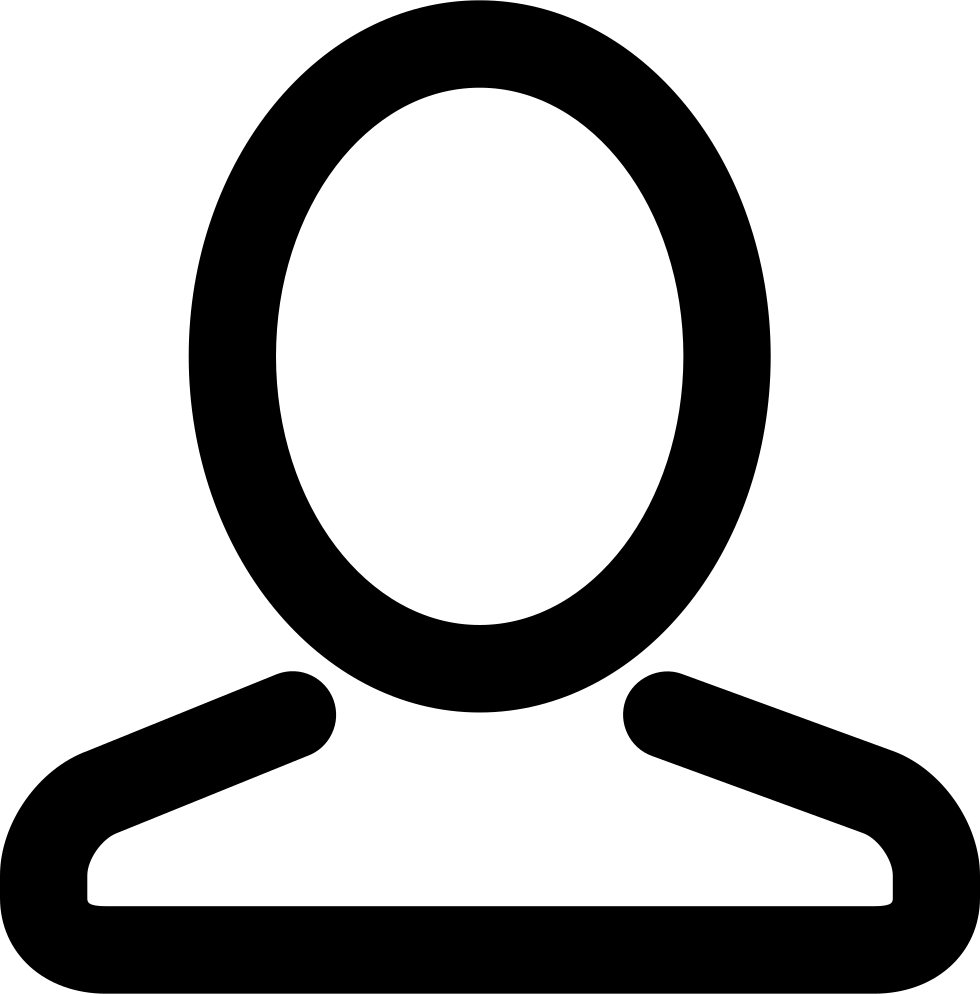
Introduction:
In the world of coding challenges, there are fascinating problems that push our problem-solving skills to the limit. In this blog, we will dive into the "Maximum Product Difference Between Two Pairs" problem. We will explore the problem statement, discuss the approach, and provide a detailed implementation in both Java and Python.
Problem Statement:
Given an array of positive integers, our goal is to find the maximum possible difference between the products of any two pairs of elements from the array.
Example:
Consider the array [5, 6, 2, 7, 4]:
- The product of the two largest elements, 6 and 7, is 42.
- The product of the two smallest elements, 2 and 4, is 8. Hence, the maximum product difference is 42 - 8 = 34.
Approach:
To solve this problem, we need to identify the two largest elements and the two smallest elements from the given array. Sorting the array in ascending order allows us to access the smallest and largest elements easily.
- Sort the array in ascending order.
- Calculate the product difference as (last element * second-to-last element) - (first element * second element).
- Return the product difference as the maximum possible difference.
Java Implementation:
import java.util.Arrays;
public class MaximumProductDifference {
public static int maxProductDifference(int[] nums) {
Arrays.sort(nums);
int n = nums.length;
return (nums[n - 1] * nums[n - 2]) - (nums[0] * nums[1]);
}
public static void main(String[] args) {
int[] nums = {5, 6, 2, 7, 4};
int maxDiff = maxProductDifference(nums);
System.out.println("Maximum Product Difference: " + maxDiff);
}
}
Python Implementation:
def max_product_difference(nums):
nums.sort()
return (nums[-1] * nums[-2]) - (nums[0] * nums[1])
nums = [5, 6, 2, 7, 4]
max_diff = max_product_difference(nums)
print("Maximum Product Difference:", max_diff)
Conclusion:
Solving the "Maximum Product Difference Between Two Pairs" problem challenges us to think critically and utilize sorting techniques to identify the largest and smallest elements efficiently. By calculating the product difference, we can obtain the maximum possible difference between two pairs of elements in the given array. The implementations in Java and Python showcase the elegance and simplicity of the solution. Now armed with this knowledge, you can confidently tackle similar coding challenges and further enhance your problem-solving skills.