Mastering Zigzag Conversion: Transforming Text with Java and Python
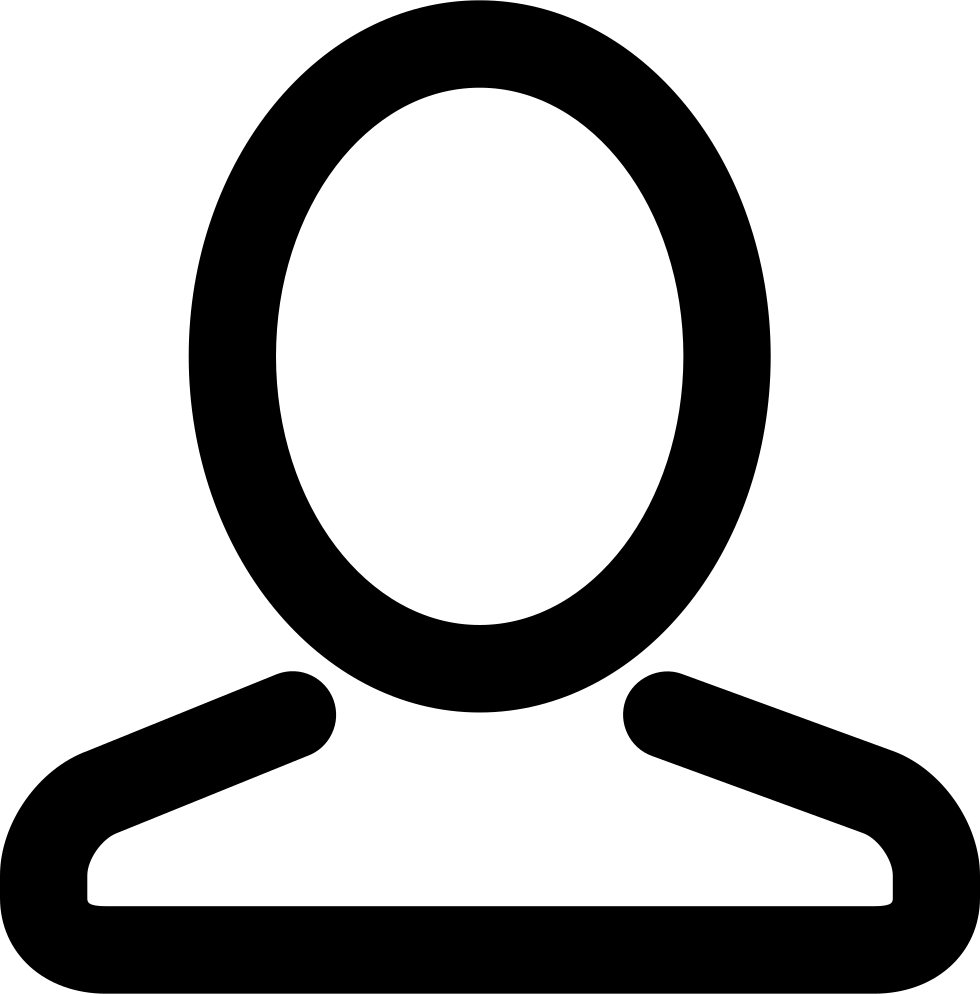
Zigzag Conversion is a fascinating text transformation technique that adds visual appeal and complexity to encoded text. If you're a Java or Python enthusiast looking to explore this algorithm, you've come to the right place! In this blog post, we'll guide you through implementing Zigzag Conversion using Java and Python, providing you with the knowledge to transform text in a captivating zigzag pattern.
-
Understanding the Zigzag Conversion: The Zigzag Conversion algorithm is designed to rearrange characters in a string, creating a visually appealing zigzag pattern. It achieves this by organizing the characters in rows, following a specific pattern of upward and downward movements. The resulting transformed text can be an intriguing visual representation of the original input.
-
How Does Zigzag Conversion Work? Let's dive into the mechanics of Zigzag Conversion. The algorithm takes two parameters: the input string and the number of rows in the zigzag pattern. It then constructs a matrix with the specified number of rows and starts filling it row by row. The characters from the input string are placed in a zigzag pattern within the matrix, following a precise traversal path.
-
Navigating the Zigzag Traversal Path: To determine the zigzag traversal path, we start at the top row and move downwards until we reach the last row. Then, we reverse direction and move diagonally upwards until we reach the top row again. This zigzag pattern continues until all characters from the input string are placed in the matrix.
-
From Matrix to Zigzag Text: Once the matrix is populated, we extract the characters row by row, concatenating them to form the transformed text. The resulting text showcases the unique zigzag pattern, adding an intriguing visual element to the original input.
-
Applications and Use Cases: The Zigzag Conversion technique finds applications in various domains. For example:
- Text compression: The transformed zigzag text can sometimes exhibit compression properties, reducing the length of the original text.
- Visual representation: The zigzag pattern can be visually appealing and find use in artistic representations or creative displays.
- Data encryption: The zigzag transformation can be incorporated as a step in encryption algorithms to add an extra layer of complexity.
-
Implementing Zigzag Conversion: Implementing Zigzag Conversion can be a fun coding exercise. By understanding the traversal path and matrix manipulation, you can develop your own algorithm or explore existing implementations in programming languages like Python, Java, or JavaScript.
Java Implementation:
public String zigzagConversion(String input, int numRows) {
if (numRows <= 1 || input.length() <= numRows)
return input;
StringBuilder[] rows = new StringBuilder[numRows];
for (int i = 0; i < numRows; i++) {
rows[i] = new StringBuilder();
}
int currRow = 0;
boolean goingDown = false;
for (char c : input.toCharArray()) {
rows[currRow].append(c);
if (currRow == 0 || currRow == numRows - 1) {
goingDown = !goingDown;
}
currRow += goingDown ? 1 : -1;
}
StringBuilder result = new StringBuilder();
for (StringBuilder row : rows) {
result.append(row);
}
return result.toString();
}
Python Implementation:
def zigzag_conversion(input, numRows):
if numRows <= 1 or len(input) <= numRows:
return input
rows = [''] * numRows
currRow = 0
goingDown = False
for c in input:
rows[currRow] += c
if currRow == 0 or currRow == numRows - 1:
goingDown = not goingDown
currRow += 1 if goingDown else -1
return ''.join(rows)
Conclusion:
By mastering Zigzag Conversion in Java and Python, you now have the ability to transform plain text into captivating zigzag patterns. This algorithm opens doors to creative text visualization, data compression possibilities, and encryption techniques. So go ahead, implement Zigzag Conversion using the provided code examples, and unlock a new dimension of text transformation!
Note: Remember to test your code thoroughly, experiment with different inputs, and enjoy the beauty of Zigzag Conversion. Happy coding!