Find children of a given binary tree by target node or by target value
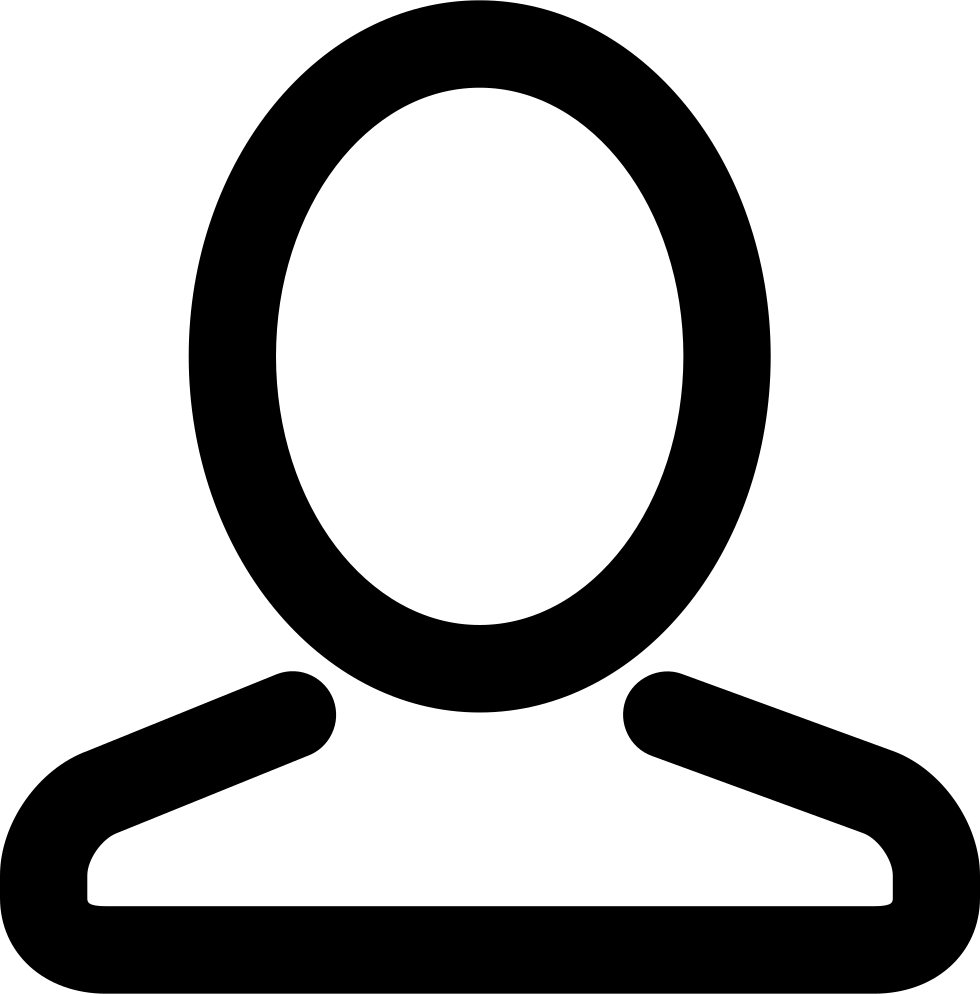
Using Target Node:
To print the children of a given binary tree node in Java, you can use a recursive approach. Here's an example code snippet that demonstrates this:
class Node {
int data;
Node left, right;
public Node(int item) {
data = item;
left = right = null;
}
}
class BinaryTree {
Node root;
public BinaryTree() {
root = null;
}
// Function to print the children of a given node
void printChildren(Node node) {
if (node == null) {
System.out.println("Node is null");
return;
}
if (node.left != null)
System.out.println("Left child: " + node.left.data);
else
System.out.println("No left child");
if (node.right != null)
System.out.println("Right child: " + node.right.data);
else
System.out.println("No right child");
}
public static void main(String[] args) {
BinaryTree tree = new BinaryTree();
tree.root = new Node(1);
tree.root.left = new Node(2);
tree.root.right = new Node(3);
tree.root.left.left = new Node(4);
tree.root.left.right = new Node(5);
Node targetNode = tree.root.left;
tree.printChildren(targetNode);
}
}
In this example, the Node
class represents a binary tree node with a data value and references to the left and right child nodes. The BinaryTree
class has a method printChildren
that takes a node as input and prints its left and right children if they exist.
In the main
method, we create a binary tree and assign values to its nodes. Then, we define a targetNode
variable that represents the node for which we want to print the children. Finally, we call printChildren
method with targetNode
as an argument to print its children.
Using Target Node Value:
To print the children of a given binary tree node value in Java, you can modify the previous code snippet to search for the node with the specified value and then print its children. Here's an updated version of the code:
class Node {
int data;
Node left, right;
public Node(int item) {
data = item;
left = right = null;
}
}
class BinaryTree {
Node root;
public BinaryTree() {
root = null;
}
// Function to search for a node with a given value
Node searchNode(Node node, int value) {
if (node == null || node.data == value)
return node;
// Recursively search in the left and right subtrees
Node leftResult = searchNode(node.left, value);
if (leftResult != null)
return leftResult;
Node rightResult = searchNode(node.right, value);
if (rightResult != null)
return rightResult;
return null;
}
// Function to print the children of a given node
void printChildren(int value) {
Node targetNode = searchNode(root, value);
if (targetNode == null) {
System.out.println("Node with value " + value + " not found");
return;
}
if (targetNode.left != null)
System.out.println("Left child: " + targetNode.left.data);
else
System.out.println("No left child");
if (targetNode.right != null)
System.out.println("Right child: " + targetNode.right.data);
else
System.out.println("No right child");
}
public static void main(String[] args) {
BinaryTree tree = new BinaryTree();
tree.root = new Node(1);
tree.root.left = new Node(2);
tree.root.right = new Node(3);
tree.root.left.left = new Node(4);
tree.root.left.right = new Node(5);
int targetValue = 2;
tree.printChildren(targetValue);
}
}
In this updated code, we added a new method searchNode
that recursively searches for a node with the specified value in the binary tree. It returns the node if found, or null
otherwise.
In the printChildren
method, we first call searchNode
to find the node with the given value. If the node is found, we print its left and right children (if they exist) as before. If the node is not found, we print a message indicating that the node was not found.
In the main
method, we create a binary tree and assign values to its nodes. Then, we define a targetValue
variable that represents the value of the node for which we want to print the children. Finally, we call printChildren
method with targetValue
as an argument to print the children of the node with that value.