Exploring the Elegance of Binary Tree Inorder Traversal: Unveiling its Implementation in Java and Python
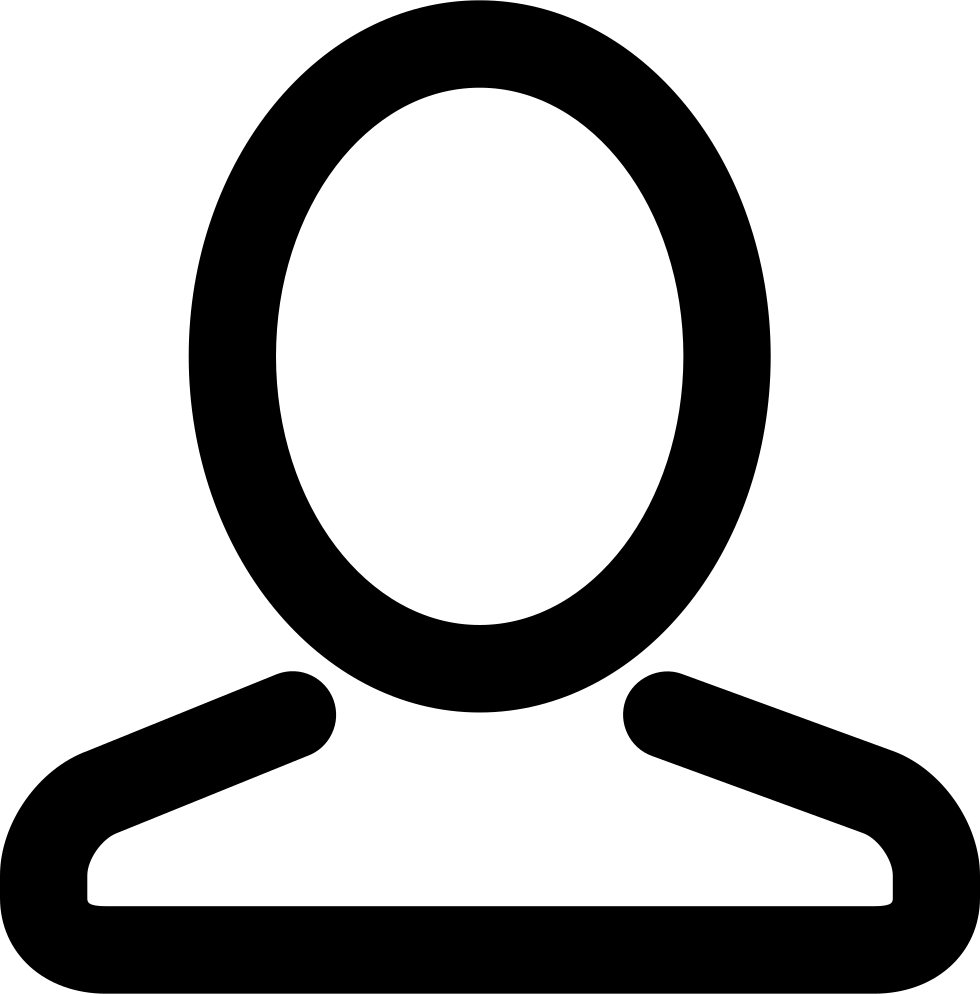
Binary tree traversal is a fundamental operation that allows us to visit and process every node in a binary tree. Among the various traversal techniques, inorder traversal holds a special place. In this blog, we will embark on a journey to explore the elegance of binary tree inorder traversal, understand its significance, and provide implementation examples in both Java and Python.
Understanding Binary Tree Inorder Traversal:
In binary tree inorder traversal, we visit the nodes of a binary tree in a specific order. The order followed is: left subtree, root node, and then the right subtree. This traversal technique is named "inorder" as it visits the nodes in the order they would appear in the sorted output of the tree.
The Beauty of Inorder Traversal:
Binary tree inorder traversal offers a multitude of benefits. It allows us to retrieve the elements of a binary search tree in ascending order, making it a crucial technique for data retrieval and sorting. Additionally, inorder traversal can be used to perform various operations on binary trees, such as evaluating expressions, creating a copy of the tree, or building an expression in infix notation.
Implementation in Java:
Let's explore a Java implementation of binary tree inorder traversal using the iterative approach and stack data structure.
import java.util.*;
class TreeNode {
int val;
TreeNode left;
TreeNode right;
TreeNode(int val) {
this.val = val;
}
}
public class BinaryTreeInorderTraversal {
public List<Integer> inorderTraversal(TreeNode root) {
List<Integer> result = new ArrayList<>();
Stack<TreeNode> stack = new Stack<>();
TreeNode current = root;
while (current != null || !stack.isEmpty()) {
while (current != null) {
stack.push(current);
current = current.left;
}
current = stack.pop();
result.add(current.val);
current = current.right;
}
return result;
}
public static void main(String[] args) {
BinaryTreeInorderTraversal solution = new BinaryTreeInorderTraversal();
// Create a sample binary tree
TreeNode root = new TreeNode(1);
root.right = new TreeNode(2);
root.right.left = new TreeNode(3);
List<Integer> inorder = solution.inorderTraversal(root);
System.out.println("Inorder Traversal: " + inorder);
}
}
Implementation in Python:
Now, let's explore a Python implementation of binary tree inorder traversal using a recursive approach.
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
class BinaryTreeInorderTraversal:
def inorderTraversal(self, root):
result = []
def inorder(node):
if node:
inorder(node.left)
result.append(node.val)
inorder(node.right)
inorder(root)
return result
if __name__ == '__main__':
solution = BinaryTreeInorderTraversal()
# Create a sample binary tree
root = TreeNode(1)
root.right = TreeNode(2)
root.right.left = TreeNode(3)
inorder = solution.inorderTraversal(root)
print("Inorder Traversal:", inorder)
Conclusion:
Binary tree inorder traversal provides a powerful technique to visit and process nodes in a binary tree in a specific order. Its significance lies in its ability to retrieve elements in sorted order and perform various operations on binary trees. By understanding and implementing binary tree inorder traversal in Java and Python, you have expanded your knowledge and problem-solving skills in tree traversal algorithms. Embrace the elegance of inorder traversal and leverage its capabilities to handle complex tree-based problems with confidence.