Exploring Different Ways to Add Parentheses for Enhanced Arithmetic Expressions
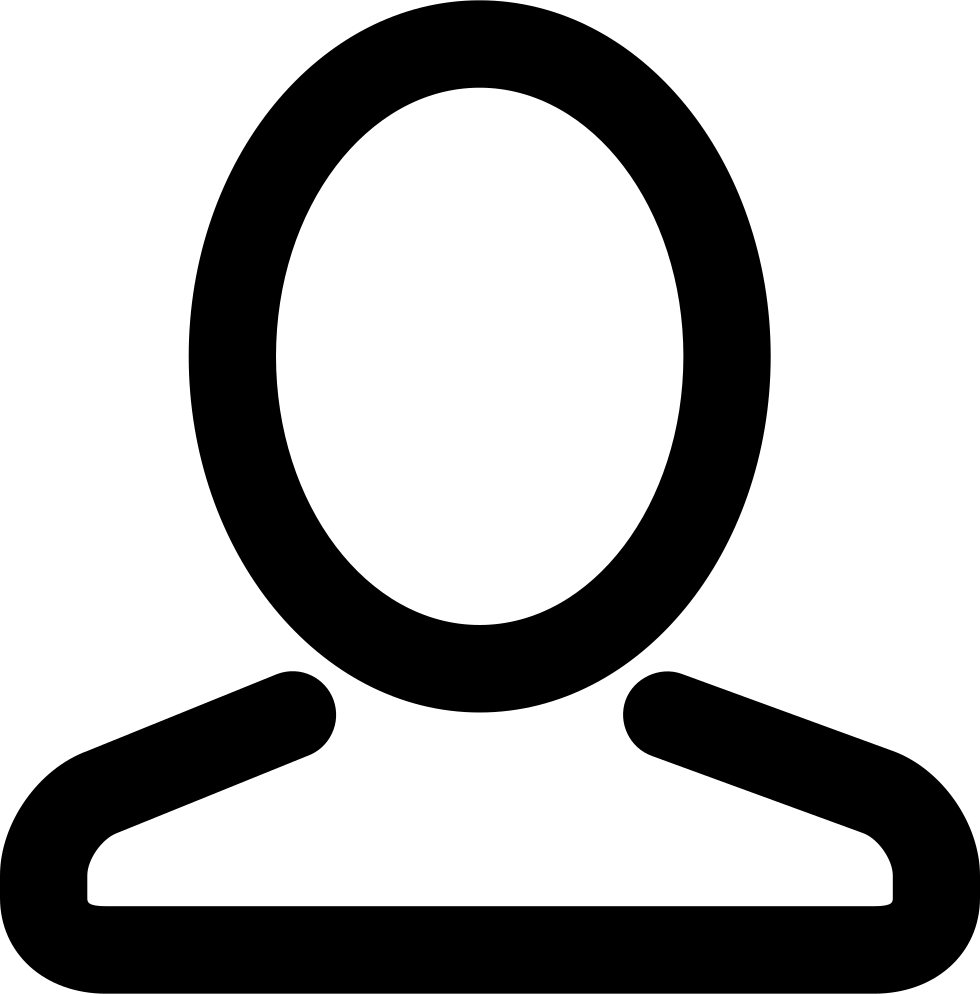
Arithmetic expressions are the backbone of mathematical computations, and adding parentheses can drastically alter their values. In this blog, we will delve into the concept of adding parentheses to arithmetic expressions and explore different strategies to maximize their impact. By understanding the various ways to add parentheses, you'll gain valuable insights into optimizing calculations and discovering new possibilities within mathematical expressions.
The Power of Parentheses:
Parentheses play a crucial role in mathematical expressions by grouping operations and altering the order of evaluation. They allow us to control the precedence of operations and introduce clarity and precision to complex calculations. By strategically adding parentheses, we can transform the outcome of an expression and unlock its full potential.
Approaches to Adding Parentheses:
There are multiple approaches to adding parentheses to arithmetic expressions, each with its unique benefits and applications. Let's explore some popular strategies:
-
Recursive Divide and Conquer:
- Divide the expression into two parts and recursively evaluate each part.
- Combine the results using different operators.
- Iterate through all possible combinations to maximize the expression's value.
-
Dynamic Programming:
- Utilize memoization to store intermediate results and avoid redundant calculations.
- Break down the expression into smaller subproblems and solve them iteratively.
- Build upon the subproblem solutions to compute the final expression value.
-
Backtracking:
- Generate all possible combinations of adding parentheses to the expression.
- Evaluate each combination and track the maximum value obtained.
- Use backtracking to explore all possible paths and find the optimal expression.
Implementation Examples:
Let's take a look at two implementation examples in Python to demonstrate different ways of adding parentheses.
1. Recursive Approach:
def diffWaysToCompute(expression):
if expression.isdigit():
return [int(expression)]
result = []
for i in range(len(expression)):
if expression[i] in "+-*":
left = diffWaysToCompute(expression[:i])
right = diffWaysToCompute(expression[i+1:])
for l in left:
for r in right:
result.append(eval(str(l) + expression[i] + str(r)))
return result
expression = "2-1-1"
print("Different Ways to Add Parentheses:", diffWaysToCompute(expression))
2. Dynamic Programming Approach:
def diffWaysToCompute(expression):
memo = {}
def compute(expression):
if expression in memo:
return memo[expression]
if expression.isdigit():
return [int(expression)]
result = []
for i in range(len(expression)):
if expression[i] in "+-*":
left = compute(expression[:i])
right = compute(expression[i+1:])
for l in left:
for r in right:
result.append(eval(str(l) + expression[i] + str(r)))
memo[expression] = result
return result
return compute(expression)
expression = "2-1-1"
print("Different Ways to Add Parentheses:", diffWaysToCompute(expression))
Conclusion:
Adding parentheses to arithmetic expressions opens up a world of possibilities, allowing us to explore different interpretations and optimize calculations. The recursive divide and conquer, dynamic programming, and backtracking approaches provide powerful techniques to achieve this. By leveraging these strategies, you can unlock the full potential of arithmetic expressions, maximize their value, and gain new insights into mathematical computations. Start experimenting with different ways to add parentheses and witness the transformative effect on your arithmetic expressions.