Unlocking the Peak Element - A Numerical Challenge
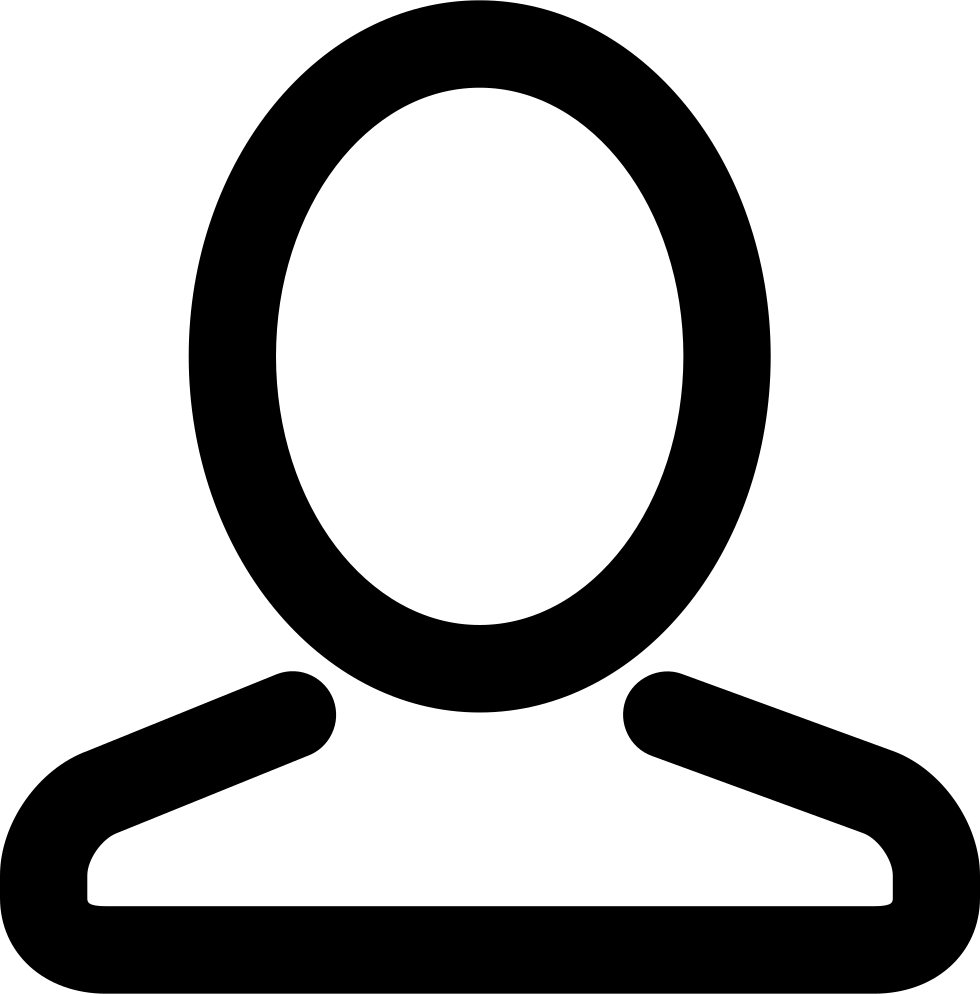
In the realm of numerical puzzles, the search for peak elements poses an intriguing challenge. In this blog, we will explore the concept of peak elements, which are defined as elements that are strictly greater than their neighbors. We will dive into the problem statement, discuss potential approaches to finding peak elements efficiently, and provide detailed implementations in both Java and Python.
Problem Statement:
Given an array of integers, our objective is to find any peak element present in the array. A peak element is defined as an element that is strictly greater than its adjacent neighbors.
Example:
Consider the array [1, 3, 2, 4, 5]. In this case, the peak elements are 3 and 5. Both elements are greater than their adjacent neighbors.
Approach:
To solve the problem of finding a peak element, we can utilize a variation of the binary search algorithm. By examining the middle element of the array and comparing it with its neighbors, we can determine whether we are in the ascending or descending portion of the array. Based on this information, we can adjust our search range accordingly until we find a peak element.
- Initialize variables for the start and end indices of the array.
- Perform a binary search until the start index is less than or equal to the end index.
- Calculate the middle index as the average of the start and end indices.
- Compare the middle element with its neighbors:
- If the middle element is greater than both its neighbors, return it as a peak element.
- If the middle element is smaller than its right neighbor, move the start index to the middle index + 1.
- If the middle element is smaller than its left neighbor, move the end index to the middle index - 1.
- Repeat steps 2-4 until a peak element is found or the search range is exhausted.
Java Implementation:
public class PeakElementFinder {
public static int findPeakElement(int[] nums) {
int start = 0;
int end = nums.length - 1;
while (start < end) {
int mid = start + (end - start) / 2;
if (nums[mid] > nums[mid + 1]) {
end = mid;
} else {
start = mid + 1;
}
}
return start;
}
public static void main(String[] args) {
int[] nums = {1, 3, 2, 4, 5};
int peakIndex = findPeakElement(nums);
int peakElement = nums[peakIndex];
System.out.println("Peak Element: " + peakElement);
}
}
Python Implementation:
def find_peak_element(nums):
start = 0
end = len(nums) - 1
while start < end:
mid = start + (end - start) // 2
if nums[mid] > nums[mid + 1]:
end = mid
else:
start = mid + 1
return start
nums = [1, 3, 2, 4, 5]
peak_index = find_peak_element(nums)
peak_element = nums[peak_index]
print("Peak Element:", peak_element)
Conclusion:
The search for peak elements, which are elements strictly greater than their neighbors, poses an interesting numerical challenge. By applying a variation of the binary search algorithm, we can efficiently locate peak elements within an array. The Java and Python implementations provided in this blog showcase the elegance and effectiveness of this approach. Armed with this knowledge, you can confidently tackle similar numerical puzzles and enhance your problem-solving skills